Reputable browser vendors issued breaking changes in late 2021 that would prevent developers from using the .alert(), .confirm(), and .prompt() methods. As a result, Salesforce moved swiftly to develop three new base components to support our continued use of these functionalities.
We can access the modules directly from within our existing Lightning Web Components. As an illustration, the alert component can be used; all we need to do is import LightningAlert from lightning/alert.
This blog will go over these three basic components (Alert, Confirm, and Prompt) and how to create notifications in a Lightning Web Component using the New Alert, Confirm, and Prompt modules.
1. lightning/alert
module
We can use the lightning/alert module to create an alert modal within our component. In cross-origin iframes, when the.alert() function is no longer supported by Chrome and Safari, LightningAlert.open() works. Instead of halting page execution like window.alert() does, LightningAlert.open() delivers a Promise. For any code that we want to run after the alert has finished, we can use async/await or.then().
This component uses the Salesforce Lightning Design System (SLDS) prompt
blueprint.
LightningAlert
supports the following attributes:
message
: Message text that displays in the alert.
label
: Header text, also used as the aria-label
. Default string is Alert
.
variant
: Two values, header
and headerless
. Default value is header
.
theme
: Color theme for the header. The theme
attribute supports the following options from SLDS:
default: white
shade: gray
inverse: dark blue
alt-inverse: darker blue
success: green
info: gray-ish blue
warning: yellow
error: red
offline: black
If an invalid value is provided, LightningAlert
uses the default
theme.
In this example we are creating an alert modal with a success message and OK button. The .open() function returns a promise that resolves when you click OK. For this we have to Import LightningAlert from the lightning/alert module in the component that we will launch the alert modal, and call LightningAlert.open() with our desired attributes.
alertNotificationLWC.html
<template>
<lightning-card title="Alert Notification" icon-name="utility:alert">
<div class="slds-p-around_medium">
<lightning-button onclick={handleAlertClick} label="Open Alert Modal">
</lightning-button>
</div>
</lightning-card>
</template>
alertNotificationLWC.js
import { LightningElement } from 'lwc';
import LightningAlert from 'lightning/alert';
export default class AlertNotificationLWC extends LightningElement {
async handleAlertClick() {
await LightningAlert.open({
message: "This is the success alert message",
theme: "success",
label: "Header for Alert"
}).then(() => {
console.log("Success Alert Closed");
});
}
}
alertNotificationLWC.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<masterLabel>Alert Notification LWC</masterLabel>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
<target>lightningCommunity__Default</target>
<target>lightningCommunity__Page</target>
</targets>
</LightningComponentBundle>
Output:-
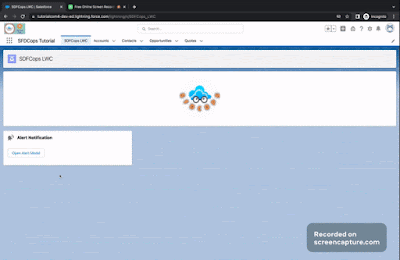
2. lightning/confirm
module
We can use the lightning/confirm module to create a confirm modal within our component. In cross-origin iframes, when the .confirm() function is no longer supported by Chrome and Safari, LightningConfirm.open() works. Instead of halting page execution like window.confirm() does, LightningConfirm.open() delivers a Promise. The .open()
function returns a promise that resolves to true when you click OK and false when you click Cancel.
This component uses the Salesforce Lightning Design System (SLDS) prompt
blueprint.
LightningConfirm
supports the same attribute as LightningAlert contains.
In this example we are creating a confirm modal with a variant: headerless and confirm button. The .confirm() function returns a promise that resolves when you click confirm button. For this we have to Import LightningConfirm from the lightning/confirm module in the component that we will launch the alert modal, and call LightningConfirm.open() with our desired attributes.
confirmNotificationLWC.html
<template>
<lightning-card title="Confirm Notification" icon-name="utility:button_choice">
<div class="slds-p-around_medium">
<lightning-button onclick={handleConfirmClick} label="Open Confirm Modal">
</lightning-button>
</div>
</lightning-card>
</template>
confirmNotificationLWC.js
import { LightningElement } from 'lwc';
import LightningConfirm from 'lightning/confirm';
export default class ConfirmNotificationLWC extends LightningElement {
async handleConfirmClick() {
const result = await LightningConfirm.open({
message: "Confirm Message",
theme: "inverse",
label: "Confirm Header",
variant: 'headerless',
});
console.log("Result is", result);
}
//result is true if OK was clicked and false if cancel was clicked
}
confirmNotificationLWC.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<masterLabel>Confirm Notification LWC</masterLabel>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
<target>lightningCommunity__Default</target>
<target>lightningCommunity__Page</target>
</targets>
</LightningComponentBundle>
Output:-
3. lightning/prompt
module
We can use the lightning/prompt module to create a prompt modal within our component. In cross-origin iframes, when the .prompt() function is no longer supported by Chrome and Safari, LightningPrompt.open() works. Instead of halting page execution like window.prompt() does. If you enter text and click OK in the prompt, the .open()
function returns a promise that resolves to the input value. If you click Cancel, the function returns a promise that resolves to null
.
This component uses the Salesforce Lightning Design System (SLDS) prompt
blueprint.
LightningAlert
supports the following attributes:
message
: Message text that displays in the alert.
defaultValue
: Optional. Initial leading text for the input text box.
label
: Header text, also used as the aria-label
. Default string is Alert
.
variant
: Two values, header
and headerless
. Default value is header
.
theme
: Color theme for the header. The theme
attribute supports the following options from SLDS:
default: white
shade: gray
inverse: dark blue
alt-inverse: darker blue
success: green
info: gray-ish blue
warning: yellow
error: red
offline: black
If an invalid value is provided, LightningAlert
uses the default
theme.
In this example we are creating a confirm modal with a header, Message and two buttons. If you enter text and click OK in the prompt, the .open()
function returns a promise that resolves to the input value. If you click Cancel, the function returns a promise that resolves to null
. For this we have to Import LightningPrompt from the lightning/prompt module in the component that we will launch the alert modal, and call LightningPrompt.open() with our desired attributes.
promptNotificationLWC.html
<template>
<lightning-card title="Prompt Notification" icon-name="utility:prompt_edit">
<div class="slds-p-around_medium">
<lightning-button onclick={handlePromptClick} label="Open Prompt Modal">
</lightning-button>
</div>
</lightning-card>
</template>
promptNotificationLWC.js
import { LightningElement } from 'lwc';
import LightningPrompt from 'lightning/prompt';
export default class PromptNotificationLWC extends LightningElement {
handlePromptClick() {
LightningPrompt.open({
message: "Prompt Message",
theme: "error",
label: "Prompt Header",
defaultValue: "Welcome to SDFCops Tutorial"
}).then((result) => {
console.log("Result", result);
});
}
}
promptNotificationLWC.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<masterLabel>Prompt Notification LWC</masterLabel>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
<target>lightningCommunity__Default</target>
<target>lightningCommunity__Page</target>
</targets>
</LightningComponentBundle>
Output:-
We hope that you find this blog helpful, if you still have queries, don’t hesitate to contact us at omsfdc91@gmail.com.
No comments:
Post a Comment